Our Spring Boot Application will provide APIs for:
- uploading File to a static folder in the Server
- downloading File from server with the link
- getting list of Files’ information (file name & url)
These are APIs to be exported:
Methods | Urls | Actions |
---|---|---|
POST | /upload | upload a File |
GET | /files | get List of Files (name & url) |
GET | /files/[filename] | download a File |
Example
Files.java
@Data
@AllArgsConstructor
public class Files {
private String name;
private String url;
}
FilesService.java
public interface FilesService {
public void init();
public void save(MultipartFile file);
public Resource load(String filename);
public void deleteAll();
public Stream<Path> loadAll();
}
FilesServiceImpl.java
Service
public class FilesServiceImpl implements FilesService {
private final Path root = Paths.get("uploads");
@Override
public void init() {
try {
Files.createDirectory(root);
} catch (IOException e) {
throw new RuntimeException("Could not initialize folder for upload!");
}
}
@Override
public void save(MultipartFile file) {
try {
Files.copy(file.getInputStream(), this.root.resolve(file.getOriginalFilename()));
} catch (Exception e) {
throw new RuntimeException("Could not store the file. Error: " + e.getMessage());
}
}
@Override
public Resource load(String filename) {
try {
Path file = root.resolve(filename);
Resource resource = new UrlResource(file.toUri());
if (resource.exists() || resource.isReadable()) {
return resource;
} else {
throw new RuntimeException("Could not read the file!");
}
} catch (MalformedURLException e) {
throw new RuntimeException("Error: " + e.getMessage());
}
}
@Override
public void deleteAll() {
FileSystemUtils.deleteRecursively(root.toFile());
}
@Override
public Stream<Path> loadAll() {
try {
return Files.walk(this.root, 1).filter(path -> !path.equals(this.root)).map(this.root::relativize);
} catch (IOException e) {
throw new RuntimeException("Could not load the files!");
}
}
}
FilesController.java
@RestController
public class FilesController {
@Autowired
FilesService storageService;
@PostMapping("/upload")
public ResponseEntity<FilesResponseMessage> uploadFile(@RequestParam("file") MultipartFile file) {
String message = "";
try {
storageService.save(file);
message = "Uploaded the file successfully: " + file.getOriginalFilename();
return ResponseEntity.status(HttpStatus.OK).body(new FilesResponseMessage(message));
} catch (Exception e) {
message = "Could not upload the file: " + file.getOriginalFilename() + "!";
return ResponseEntity.status(HttpStatus.EXPECTATION_FAILED).body(new FilesResponseMessage(message));
}
}
@GetMapping("/files")
public ResponseEntity<List<Files>> getListFiles() {
List<Files> fileInfos = storageService.loadAll().map(path -> {
String filename = path.getFileName().toString();
String url = MvcUriComponentsBuilder
.fromMethodName(FilesController.class, "getFile", path.getFileName().toString()).build().toString();
return new Files(filename, url);
}).collect(Collectors.toList());
return ResponseEntity.status(HttpStatus.OK).body(fileInfos);
}
@GetMapping("/files/{filename:.+}")
@ResponseBody
public ResponseEntity<Resource> getFile(@PathVariable String filename) {
Resource file = storageService.load(filename);
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + file.getFilename() + "\"").body(file);
}
}
FilesControllerExceptionHandler.java
@RestControllerAdvice
public class FilesControllerExceptionHandler extends ResponseEntityExceptionHandler {
@ExceptionHandler(MaxUploadSizeExceededException.class)
public ResponseEntity<FilesResponseMessage> handleMaxSizeException(MaxUploadSizeExceededException exc) {
return ResponseEntity.status(HttpStatus.EXPECTATION_FAILED).body(new FilesResponseMessage("File too large!"));
}
}
FilesResponseMessage.java
@Data
@AllArgsConstructor
public class FilesResponseMessage {
private String message;
}
Demo12Application.java
@SpringBootApplication
public class Demo12Application implements CommandLineRunner {
public static void main(String[] args) {
SpringApplication.run(Demo12Application.class, args);
}
@Autowired
FileService storageService;
@Override
public void run(String... arg) throws Exception {
storageService.deleteAll();
storageService.init();
}
}
application.properties
spring.servlet.multipart.max-file-size=500KB
spring.servlet.multipart.max-request-size=500KB
Tests
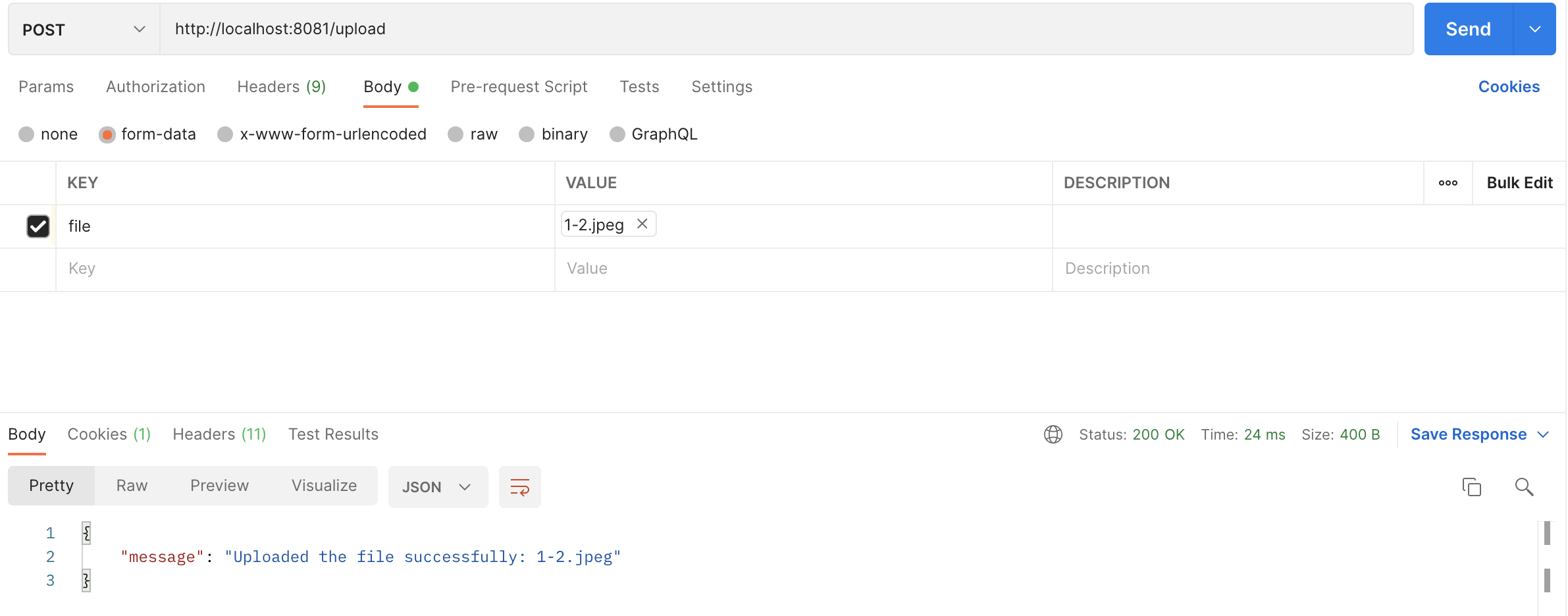
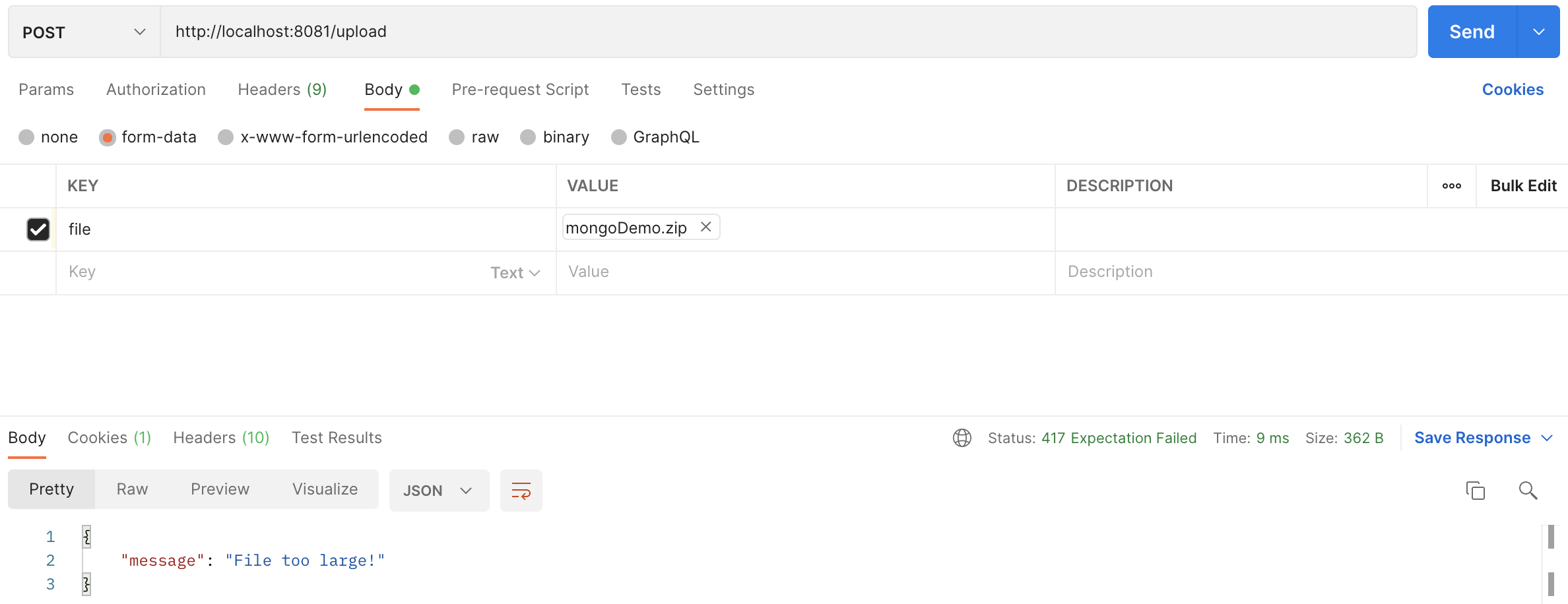
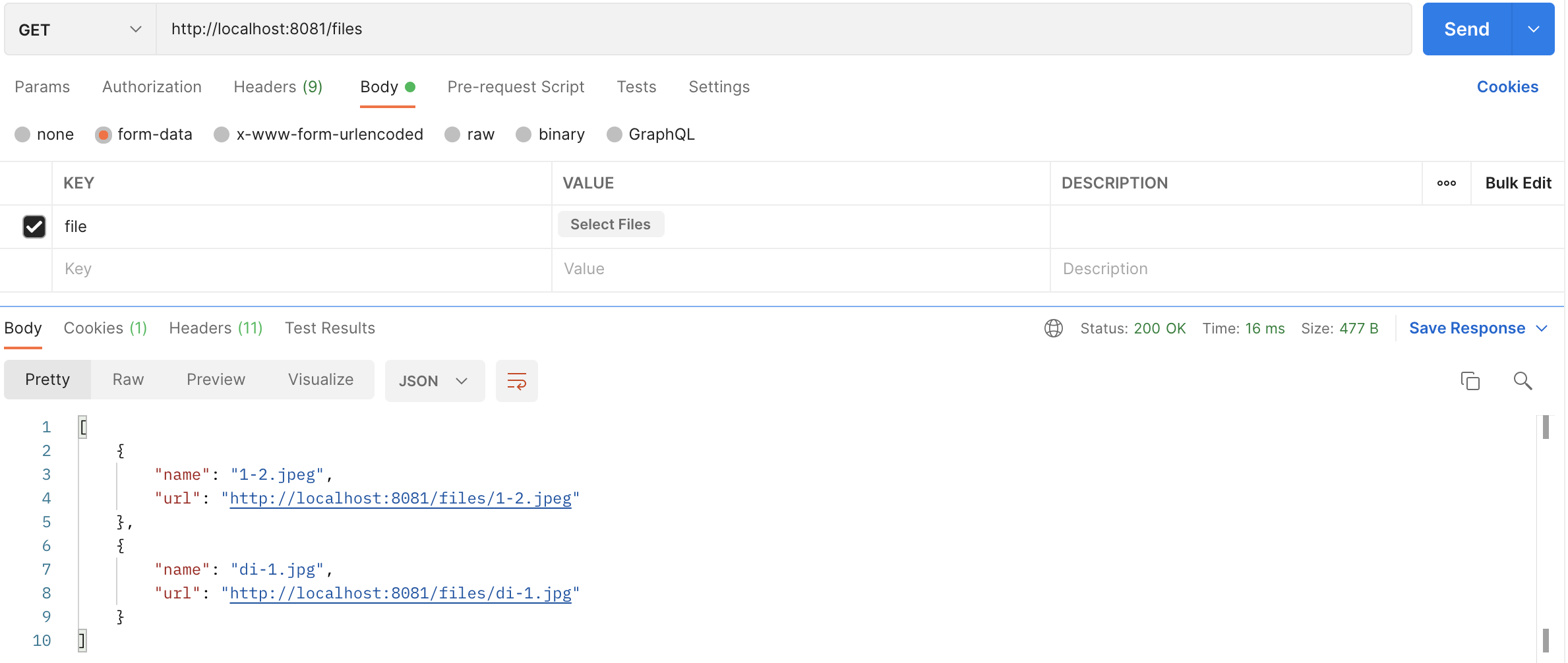
http://localhost:8081/files/di-1.jpg on browser will download the picture