We use UIViewRepresentable
to wrap the UIKit to be used in SwiftUI. Here is an example to wrap UIActivityIndicatorView
# ActivityIndicator.swift
import Foundation
import SwiftUI
struct ActivityIndicator: UIViewRepresentable {
@Binding var isAnimating: Bool
func makeUIView(context: Context) -> UIActivityIndicatorView {
let v = UIActivityIndicatorView()
return v
}
func updateUIView(_ uiView: UIActivityIndicatorView, context: Context) {
if isAnimating {
uiView.startAnimating()
} else {
uiView.stopAnimating()
}
}
typealias UIViewType = UIActivityIndicatorView
}
You only need create a file to implement the UIViewRepresentable
protocol.
And here is how you use it
# ContentView.swift
struct ContentView: View {
var body: some View {
ActivityIndicator(isAnimating: .constant(true))
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Here is the result
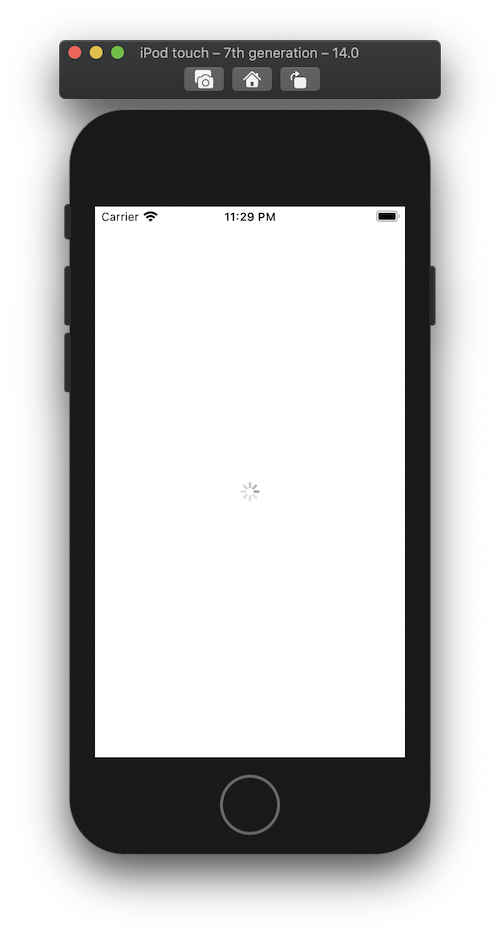